Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Create a Data Access Object for Aha Data using JDBI
A brief overview of creating a SQL Object API for Aha data in JDBI.
JDBI is a SQL convenience library for Java that exposes two different style APIs, a fluent style and a SQL object style. The CData JDBC Driver for Aha integrates connectivity to live Aha data in Java applications. By pairing these technologies, you gain simple, programmatic access to Aha data. This article walks through building a basic Data Access Object (DAO) and the accompanying code to read Aha data.
Create a DAO for the Aha Ideas Entity
The interface below declares the desired behavior for the SQL object to create a single method for each SQL statement to be implemented.
public interface MyIdeasDAO {
//request specific data from Aha (String type is used for simplicity)
@SqlQuery("SELECT Name FROM Ideas WHERE AssignedToUserId = :assignedToUserId")
String findNameByAssignedToUserId(@Bind("assignedToUserId") String assignedToUserId);
/*
* close with no args is used to close the connection
*/
void close();
}
Open a Connection to Aha
Collect the necessary connection properties and construct the appropriate JDBC URL for connecting to Aha.
Start by setting the Profile connection property to the location of the Aha! Profile on disk (e.g. C:\profiles\aha.apip). Next, set the ProfileSettings connection property to the connection string for Aha! (see below).
Aha! API Profile Settings
The Aha! API uses OAuth-based authentication.
You will first need to register an OAuth app with Aha!. This can be done from your Aha! account under 'Settings' > 'Personal' > 'Developer' > 'OAuth Applications'. Additionally, you will need to set the Domain, found in the domain name of your Aha account. For example if your Aha account is acmeinc.aha.io, then the Domain should be 'acmeinc'.
After setting the following in the connection string, you are ready to connect:
- AuthScheme: Set this to OAuth.
- InitiateOAuth: Set this to GETANDREFRESH. You can use InitiateOAuth to manage the process to obtain the OAuthAccessToken.
- OAuthClientId: Set this to the client_id that is specified in you app settings.
- OAuthClientSecret: Set this to the client_secret that is specified in you app settings.
- CallbackURL: Set this to the Redirect URI you specified in your app settings.
- Domain: Set this in the ProfileSettings to your Aha domain.
Built-in Connection String Designer
For assistance in constructing the JDBC URL, use the connection string designer built into the Aha JDBC Driver. Either double-click the JAR file or execute the jar file from the command-line.
java -jar cdata.jdbc.api.jar
Fill in the connection properties and copy the connection string to the clipboard.
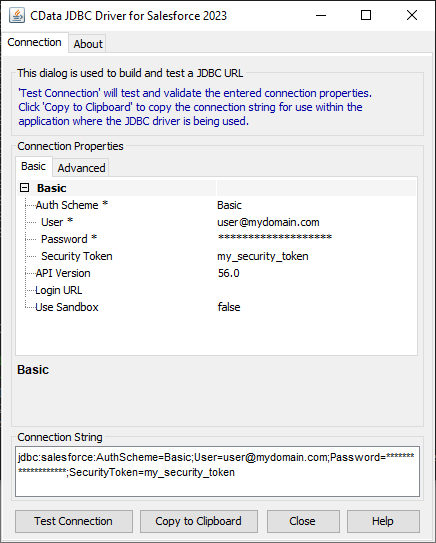
A connection string for Aha will typically look like the following:
jdbc:api:Profile=C:\profiles\aha.apip;ProfileSettings='Domain=acmeinc';Authscheme=OAuth;OAuthClientId=your_client_id;OAuthClientSecret=your_client_secret;CallbackUrl=your_callback_url;
Use the configured JDBC URL to obtain an instance of the DAO interface. The particular method shown below will open a handle bound to the instance, so the instance needs to be closed explicitly to release the handle and the bound JDBC connection.
DBI dbi = new DBI("jdbc:api:Profile=C:\profiles\aha.apip;ProfileSettings='Domain=acmeinc';Authscheme=OAuth;OAuthClientId=your_client_id;OAuthClientSecret=your_client_secret;CallbackUrl=your_callback_url;");
MyIdeasDAO dao = dbi.open(MyIdeasDAO.class);
//do stuff with the DAO
dao.close();
Read Aha Data
With the connection open to Aha, simply call the previously defined method to retrieve data from the Ideas entity in Aha.
//disply the result of our 'find' method
String name = dao.findNameByAssignedToUserId("my_user_id");
System.out.println(name);
Since the JDBI library is able to work with JDBC connections, you can easily produce a SQL Object API for Aha by integrating with the CData JDBC Driver for Aha. Download a free trial and work with live Aha data in custom Java applications today.