Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →How to Visualize Email Data in Python with pandas
Use pandas and other modules to analyze and visualize live Email data in Python.
The rich ecosystem of Python modules lets you get to work quickly and integrate your systems more effectively. With the CData Python Connector for Email, the pandas & Matplotlib modules, and the SQLAlchemy toolkit, you can build Email-connected Python applications and scripts for visualizing Email data. This article shows how to use the pandas, SQLAlchemy, and Matplotlib built-in functions to connect to Email data, execute queries, and visualize the results.
With built-in optimized data processing, the CData Python Connector offers unmatched performance for interacting with live Email data in Python. When you issue complex SQL queries from Email, the driver pushes supported SQL operations, like filters and aggregations, directly to Email and utilizes the embedded SQL engine to process unsupported operations client-side (often SQL functions and JOIN operations).
Connecting to Email Data
Connecting to Email data looks just like connecting to any relational data source. Create a connection string using the required connection properties. For this article, you will pass the connection string as a parameter to the create_engine function.
The User and Password properties, under the Authentication section, must be set to valid credentials. The Server must be specified to retrieve emails and the SMTPServer must be specified to send emails.
Follow the procedure below to install the required modules and start accessing Email through Python objects.
Install Required Modules
Use the pip utility to install the pandas & Matplotlib modules and the SQLAlchemy toolkit:
pip install pandas pip install matplotlib pip install sqlalchemy
Be sure to import the module with the following:
import pandas import matplotlib.pyplot as plt from sqlalchemy import create_engine
Visualize Email Data in Python
You can now connect with a connection string. Use the create_engine function to create an Engine for working with Email data.
engine = create_engine("email:///[email protected]&Password=password&Server=imap.gmail.com&Port=993&SMTP Server=smtp.gmail.com&SMTP Port=465&SSL Mode=EXPLICIT&Protocol=IMAP&Mailbox=Inbox")
Execute SQL to Email
Use the read_sql function from pandas to execute any SQL statement and store the resultset in a DataFrame.
df = pandas.read_sql("SELECT Mailbox, RecentMessagesCount FROM Mailboxes WHERE Mailbox = 'Spam'", engine)
Visualize Email Data
With the query results stored in a DataFrame, use the plot function to build a chart to display the Email data. The show method displays the chart in a new window.
df.plot(kind="bar", x="Mailbox", y="RecentMessagesCount") plt.show()
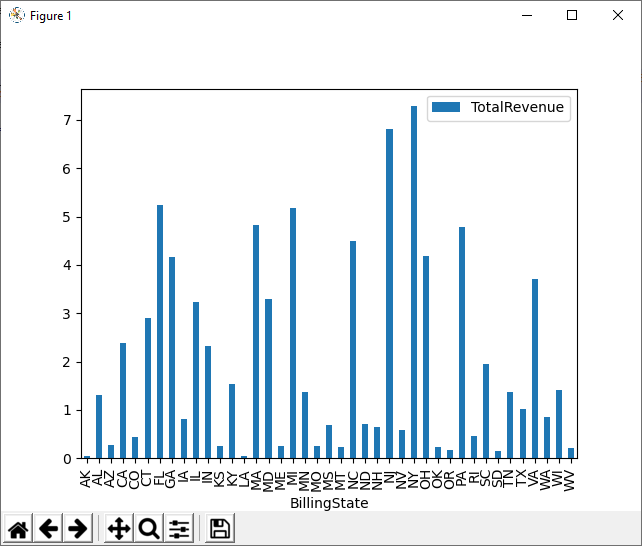
Free Trial & More Information
Download a free, 30-day trial of the CData Python Connector for Email to start building Python apps and scripts with connectivity to Email data. Reach out to our Support Team if you have any questions.
Full Source Code
import pandas import matplotlib.pyplot as plt from sqlalchemy import create_engin engine = create_engine("email:///[email protected]&Password=password&Server=imap.gmail.com&Port=993&SMTP Server=smtp.gmail.com&SMTP Port=465&SSL Mode=EXPLICIT&Protocol=IMAP&Mailbox=Inbox") df = pandas.read_sql("SELECT Mailbox, RecentMessagesCount FROM Mailboxes WHERE Mailbox = 'Spam'", engine) df.plot(kind="bar", x="Mailbox", y="RecentMessagesCount") plt.show()