Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →DataBind Charts to Google Cloud Storage Data
Use the standard ADO.NET procedures for databinding to provide bidirectional access to Google Cloud Storage data from controls in the Visual Studio toolbox. This article demonstrates a graphical approach using wizards in Visual Studio, as well as how to databind with only a few lines of code.
DataBinding facilitates two-way interaction with data through UI controls. Using the CData ADO.NET Provider for Google Cloud Storage streamlines the process of binding Google Cloud Storage data to Windows Forms and Web controls within Visual Studio. In this article, we will demonstrate using wizards to establish a binding between Google Cloud Storage data and a chart that dynamically updates. Additionally, the code walk-through section will guide you through the creation of a chart using just 10 lines of code.
Binding Data to a Chart
DataBinding to a Chart consists of three steps: Instantiate the control, configure the data source, and databind.
Configure the Connection and Select Database Objects
To create a chart control and establish a connection to Google Cloud Storage, follow the steps outlined below using the Data Source Configuration Wizard. Within the wizard, you'll have the option to choose the specific Google Cloud Storage entities you wish to bind to.
- In a Windows Forms project, drag and drop a Chart control from the toolbox to the form. In the Data section of the Chart properties, select DataSource and then select Add Project Data Source from the menu.
- In the Data Source Configuration Wizard that appears, select Database -> Dataset.
- In the Choose Your Data Connection step, click New Connection.
In the Add Connection dialog, click Change to select the CData Google Cloud Storage Data Source.
Below is a typical connection string:
ProjectId='project1';InitiateOAuth=GETANDREFRESH
Authenticate with a User Account
You can connect without setting any connection properties for your user credentials. After setting InitiateOAuth to GETANDREFRESH, you are ready to connect.
When you connect, the Google Cloud Storage OAuth endpoint opens in your default browser. Log in and grant permissions, then the OAuth process completes
Authenticate with a Service Account
Service accounts have silent authentication, without user authentication in the browser. You can also use a service account to delegate enterprise-wide access scopes.
You need to create an OAuth application in this flow. See the Help documentation for more information. After setting the following connection properties, you are ready to connect:
- InitiateOAuth: Set this to GETANDREFRESH.
- OAuthJWTCertType: Set this to "PFXFILE".
- OAuthJWTCert: Set this to the path to the .p12 file you generated.
- OAuthJWTCertPassword: Set this to the password of the .p12 file.
- OAuthJWTCertSubject: Set this to "*" to pick the first certificate in the certificate store.
- OAuthJWTIssuer: In the service accounts section, click Manage Service Accounts and set this field to the email address displayed in the service account Id field.
- OAuthJWTSubject: Set this to your enterprise Id if your subject type is set to "enterprise" or your app user Id if your subject type is set to "user".
- ProjectId: Set this to the Id of the project you want to connect to.
The OAuth flow for a service account then completes.
When you configure the connection, you may also want to set the Max Rows connection property. This will limit the number of rows returned, which is especially helpful for improving performance when designing reports and visualizations.
- Choose the database objects you want to work with. This example uses the Buckets table.
DataBind
After adding the data source and selecting database objects, you can bind the objects to the chart. This example assigns the x-axis to Name and the y-axis to OwnerId.
- In the Chart properties, click the button in the Series property to open the Series Collection Editor.
- In the Series properties, select the columns you want for the x- and y-axes: Select columns from the menu in the XValueMember and YValueMember properties.
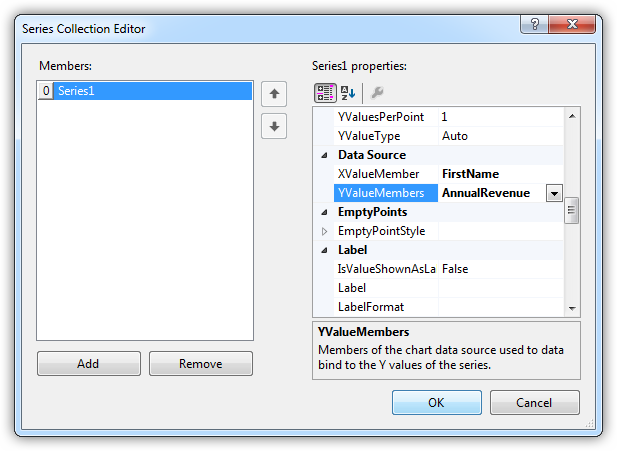
The chart is now databound to the Google Cloud Storage data. Run the chart to display the current data.
Code Walk-through
DataBinding to Google Cloud Storage data requires only a few lines of code and can be completed in three easy steps.
- Connect to Google Cloud Storage.
- Create the GoogleCloudStorageDataAdapter to execute the query and create a DataSet to be filled with its results.
- DataBind the result set to the chart.
Below is the complete code:
GoogleCloudStorageConnection conn = new GoogleCloudStorageConnection("ProjectId='project1';InitiateOAuth=GETANDREFRESH");
GoogleCloudStorageCommand comm = new GoogleCloudStorageCommand("SELECT Name, OwnerId FROM Buckets WHERE Name = 'TestBucket'", conn);
GoogleCloudStorageDataAdapter da = new GoogleCloudStorageDataAdapter(comm);
DataSet dataset = new DataSet();
da.Fill(dataset);
chart1.DataSource = dataset;
chart1.Series[0].XValueMember = "Name";
chart1.Series[0].YValueMembers = "OwnerId";
// Insert code for additional chart formatting here.
chart1.DataBind();