Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →DataBind Charts to Paylocity Data
Use the standard ADO.NET procedures for databinding to provide bidirectional access to Paylocity data from controls in the Visual Studio toolbox. This article demonstrates a graphical approach using wizards in Visual Studio, as well as how to databind with only a few lines of code.
DataBinding facilitates two-way interaction with data through UI controls. Using the CData ADO.NET Provider for Paylocity streamlines the process of binding Paylocity data to Windows Forms and Web controls within Visual Studio. In this article, we will demonstrate using wizards to establish a binding between Paylocity data and a chart that dynamically updates. Additionally, the code walk-through section will guide you through the creation of a chart using just 10 lines of code.
Binding Data to a Chart
DataBinding to a Chart consists of three steps: Instantiate the control, configure the data source, and databind.
Configure the Connection and Select Database Objects
To create a chart control and establish a connection to Paylocity, follow the steps outlined below using the Data Source Configuration Wizard. Within the wizard, you'll have the option to choose the specific Paylocity entities you wish to bind to.
- In a Windows Forms project, drag and drop a Chart control from the toolbox to the form. In the Data section of the Chart properties, select DataSource and then select Add Project Data Source from the menu.
- In the Data Source Configuration Wizard that appears, select Database -> Dataset.
- In the Choose Your Data Connection step, click New Connection.
In the Add Connection dialog, click Change to select the CData Paylocity Data Source.
Below is a typical connection string:
OAuthClientID=YourClientId;OAuthClientSecret=YourClientSecret;RSAPublicKey=YourRSAPubKey;Key=YourKey;IV=YourIV;InitiateOAuth=GETANDREFRESH
Set the following to establish a connection to Paylocity:
- RSAPublicKey: Set this to the RSA Key associated with your Paylocity, if the RSA Encryption is enabled in the Paylocity account.
This property is required for executing Insert and Update statements, and it is not required if the feature is disabled.
- UseSandbox: Set to true if you are using sandbox account.
- CustomFieldsCategory: Set this to the Customfields category. This is required when IncludeCustomFields is set to true. The default value for this property is PayrollAndHR.
- Key: The AES symmetric key(base 64 encoded) encrypted with the Paylocity Public Key. It is the key used to encrypt the content.
Paylocity will decrypt the AES key using RSA decryption.
It is an optional property if the IV value not provided, The driver will generate a key internally. - IV: The AES IV (base 64 encoded) used when encrypting the content. It is an optional property if the Key value not provided, The driver will generate an IV internally.
Connect Using OAuth Authentication
You must use OAuth to authenticate with Paylocity. OAuth requires the authenticating user to interact with Paylocity using the browser. For more information, refer to the OAuth section in the Help documentation.
The Pay Entry API
The Pay Entry API is completely separate from the rest of the Paylocity API. It uses a separate Client ID and Secret, and must be explicitly requested from Paylocity for access to be granted for an account. The Pay Entry API allows you to automatically submit payroll information for individual employees, and little else. Due to the extremely limited nature of what is offered by the Pay Entry API, we have elected not to give it a separate schema, but it may be enabled via the UsePayEntryAPI connection property.
Please be aware that when setting UsePayEntryAPI to true, you may only use the CreatePayEntryImportBatch & MergePayEntryImportBatchgtable stored procedures, the InputTimeEntry table, and the OAuth stored procedures. Attempts to use other features of the product will result in an error. You must also store your OAuthAccessToken separately, which often means setting a different OAuthSettingsLocation when using this connection property.
When you configure the connection, you may also want to set the Max Rows connection property. This will limit the number of rows returned, which is especially helpful for improving performance when designing reports and visualizations.
- RSAPublicKey: Set this to the RSA Key associated with your Paylocity, if the RSA Encryption is enabled in the Paylocity account.
- Choose the database objects you want to work with. This example uses the Employee table.
DataBind
After adding the data source and selecting database objects, you can bind the objects to the chart. This example assigns the x-axis to FirstName and the y-axis to LastName.
- In the Chart properties, click the button in the Series property to open the Series Collection Editor.
- In the Series properties, select the columns you want for the x- and y-axes: Select columns from the menu in the XValueMember and YValueMember properties.
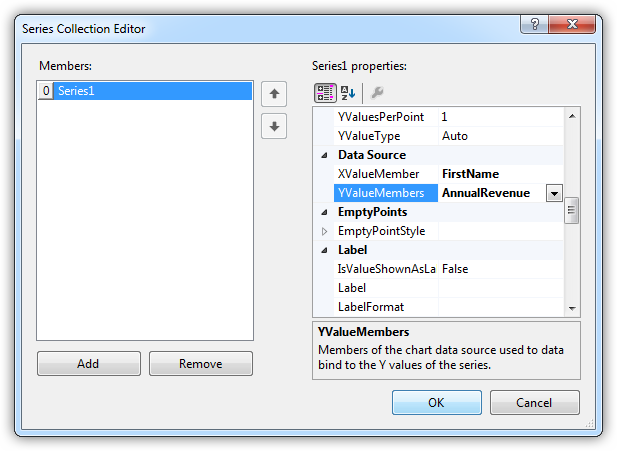
The chart is now databound to the Paylocity data. Run the chart to display the current data.
Code Walk-through
DataBinding to Paylocity data requires only a few lines of code and can be completed in three easy steps.
- Connect to Paylocity.
- Create the PaylocityDataAdapter to execute the query and create a DataSet to be filled with its results.
- DataBind the result set to the chart.
Below is the complete code:
PaylocityConnection conn = new PaylocityConnection("OAuthClientID=YourClientId;OAuthClientSecret=YourClientSecret;RSAPublicKey=YourRSAPubKey;Key=YourKey;IV=YourIV;InitiateOAuth=GETANDREFRESH");
PaylocityCommand comm = new PaylocityCommand("SELECT FirstName, LastName FROM Employee WHERE EmployeeId = '1234'", conn);
PaylocityDataAdapter da = new PaylocityDataAdapter(comm);
DataSet dataset = new DataSet();
da.Fill(dataset);
chart1.DataSource = dataset;
chart1.Series[0].XValueMember = "FirstName";
chart1.Series[0].YValueMembers = "LastName";
// Insert code for additional chart formatting here.
chart1.DataBind();