Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Create a Data Access Object for Salesforce Marketing Data using JDBI
A brief overview of creating a SQL Object API for Salesforce Marketing data in JDBI.
JDBI is a SQL convenience library for Java that exposes two different style APIs, a fluent style and a SQL object style. The CData JDBC Driver for Salesforce Marketing integrates connectivity to live Salesforce Marketing data in Java applications. By pairing these technologies, you gain simple, programmatic access to Salesforce Marketing data. This article walks through building a basic Data Access Object (DAO) and the accompanying code to read and write Salesforce Marketing data.
Create a DAO for the Salesforce Marketing Subscriber Entity
The interface below declares the desired behavior for the SQL object to create a single method for each SQL statement to be implemented.
public interface MySubscriberDAO {
//insert new data into Salesforce Marketing
@SqlUpdate("INSERT INTO Subscriber (EmailAddress, Status) values (:emailAddress, :status)")
void insert(@Bind("emailAddress") String emailAddress, @Bind("status") String status);
//request specific data from Salesforce Marketing (String type is used for simplicity)
@SqlQuery("SELECT Status FROM Subscriber WHERE EmailAddress = :emailAddress")
String findStatusByEmailAddress(@Bind("emailAddress") String emailAddress);
/*
* close with no args is used to close the connection
*/
void close();
}
Open a Connection to Salesforce Marketing
Collect the necessary connection properties and construct the appropriate JDBC URL for connecting to Salesforce Marketing.
Authenticating to the Salesforce Marketing Cloud APIs
Set the User and Password to your login credentials, or to the credentials for a sandbox user if you are connecting to a sandbox account.
Connecting to the Salesforce Marketing Cloud APIs
By default, the data provider connects to production environments. Set UseSandbox to true to use a Salesforce Marketing Cloud sandbox account.
The default Instance is s7 of the Web Services API; however, if you use a different instance, you can set Instance.
Built-in Connection String Designer
For assistance in constructing the JDBC URL, use the connection string designer built into the Salesforce Marketing JDBC Driver. Either double-click the JAR file or execute the jar file from the command-line.
java -jar cdata.jdbc.sfmarketingcloud.jar
Fill in the connection properties and copy the connection string to the clipboard.
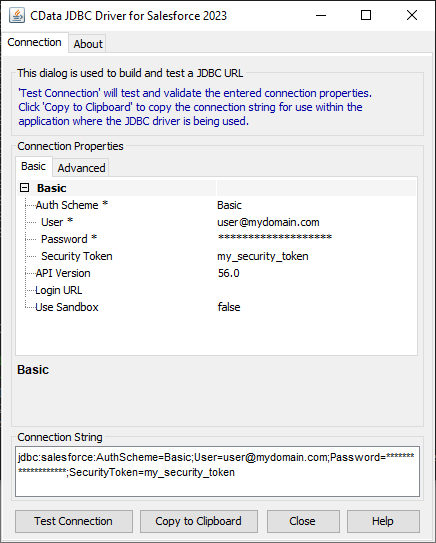
A connection string for Salesforce Marketing will typically look like the following:
jdbc:sfmarketingcloud:User=myUser;Password=myPassword;InitiateOAuth=GETANDREFRESH
Use the configured JDBC URL to obtain an instance of the DAO interface. The particular method shown below will open a handle bound to the instance, so the instance needs to be closed explicitly to release the handle and the bound JDBC connection.
DBI dbi = new DBI("jdbc:sfmarketingcloud:User=myUser;Password=myPassword;InitiateOAuth=GETANDREFRESH");
MySubscriberDAO dao = dbi.open(MySubscriberDAO.class);
//do stuff with the DAO
dao.close();
Read Salesforce Marketing Data
With the connection open to Salesforce Marketing, simply call the previously defined method to retrieve data from the Subscriber entity in Salesforce Marketing.
//disply the result of our 'find' method
String status = dao.findStatusByEmailAddress("[email protected]");
System.out.println(status);
Write Salesforce Marketing Data
It is also simple to write data to Salesforce Marketing, using the previously defined method.
//add a new entry to the Subscriber entity
dao.insert(newEmailAddress, newStatus);
Since the JDBI library is able to work with JDBC connections, you can easily produce a SQL Object API for Salesforce Marketing by integrating with the CData JDBC Driver for Salesforce Marketing. Download a free trial and work with live Salesforce Marketing data in custom Java applications today.