Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →DataBind Charts to SAP Ariba Source Data
Use the standard ADO.NET procedures for databinding to provide bidirectional access to SAP Ariba Source data from controls in the Visual Studio toolbox. This article demonstrates a graphical approach using wizards in Visual Studio, as well as how to databind with only a few lines of code.
DataBinding facilitates two-way interaction with data through UI controls. Using the CData ADO.NET Provider for SAP Ariba Source streamlines the process of binding SAP Ariba Source data to Windows Forms and Web controls within Visual Studio. In this article, we will demonstrate using wizards to establish a binding between SAP Ariba Source data and a chart that dynamically updates. Additionally, the code walk-through section will guide you through the creation of a chart using just 10 lines of code.
DataBind to a Chart
DataBinding consists of three steps: Instantiate the control, configure the data source, and databind.
Configure the Connection and Select Database Objects
To create a chart control and establish a connection to SAP Ariba Source, follow the steps outlined below using the Data Source Configuration Wizard. Within the wizard, you'll have the option to choose the specific SAP Ariba Source entities you wish to bind to.
- In a Windows Forms project, drag and drop a Chart control from the toolbox to the form. In the Data section of the Chart properties, select DataSource and then select Add Project Data Source from the menu.
- In the Data Source Configuration Wizard that appears, select Database -> Dataset.
- In the Choose Your Data Connection step, click New Connection.
In the Add Connection dialog, click Change to select the CData SAP Ariba Source Data Source.
Below is a typical connection string:
API=SupplierDataAPIWithPagination-V4;APIKey=wWVLn7WTAXrIRMAzZ6VnuEj7Ekot5jnU;Environment=SANDBOX;Realm=testRealm;AuthScheme=OAuthClient;InitiateOAuth=GETANDREFRESH
In order to connect with SAP Ariba Source, set the following:
- API: Specify which API you would like the provider to retrieve SAP Ariba data from. Select the Supplier, Sourcing Project Management, or Contract API based on your business role (possible values are SupplierDataAPIWithPaginationV4, SourcingProjectManagementAPIV2, or ContractAPIV1).
- DataCenter: The data center where your account's data is hosted.
- Realm: The name of the site you want to access.
- Environment: Indicate whether you are connecting to a test or production environment (possible values are TEST or PRODUCTION).
If you are connecting to the Supplier Data API or the Contract API, additionally set the following:
- User: Id of the user on whose behalf API calls are invoked.
- PasswordAdapter: The password associated with the authenticating User.
If you're connecting to the Supplier API, set ProjectId to the Id of the sourcing project you want to retrieve data from.
Authenticating with OAuth
After setting connection properties, you need to configure OAuth connectivity to authenticate.
- Set AuthScheme to OAuthClient.
- Register an application with the service to obtain the APIKey, OAuthClientId and OAuthClientSecret.
For more information on creating an OAuth application, refer to the Help documentation.
Automatic OAuth
After setting the following, you are ready to connect:
-
APIKey: The Application key in your app settings.
OAuthClientId: The OAuth Client Id in your app settings.
OAuthClientSecret: The OAuth Secret in your app settings.
When you connect, the provider automatically completes the OAuth process:
- The provider obtains an access token from SAP Ariba and uses it to request data.
- The provider refreshes the access token automatically when it expires.
- The OAuth values are saved in memory relative to the location specified in OAuthSettingsLocation.
When you configure the connection, you may also want to set the Max Rows connection property. This will limit the number of rows returned, which is especially helpful for improving performance when designing reports and visualizations.
- Choose the database objects you want to work with. This example uses the Vendors table.
DataBind
After adding the data source and selecting database objects, you can bind the objects to the chart. This example assigns the x-axis to SMVendorID and the y-axis to Category.
- In the Chart properties, click the button in the Series property to open the Series Collection Editor.
- In the Series properties, select the columns you want for the x- and y-axes: Select columns from the menu in the XValueMember and YValueMember properties.
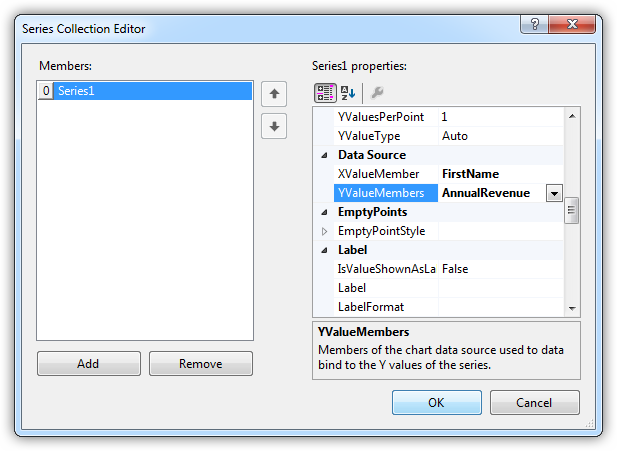
The chart is now databound to the SAP Ariba Source data. Run the chart to display the current data.
Code Walk-through
DataBinding to SAP Ariba Source data requires only a few lines of code and can be completed in three easy steps.
- Connect to SAP Ariba Source.
- Create the SAPAribaSourceDataAdapter to execute the query and create a DataSet to be filled with its results.
- DataBind the result set to the chart.
Below is the complete code:
SAPAribaSourceConnection conn = new SAPAribaSourceConnection("API=SupplierDataAPIWithPagination-V4;APIKey=wWVLn7WTAXrIRMAzZ6VnuEj7Ekot5jnU;Environment=SANDBOX;Realm=testRealm;AuthScheme=OAuthClient;InitiateOAuth=GETANDREFRESH");
SAPAribaSourceCommand comm = new SAPAribaSourceCommand("SELECT SMVendorID, Category FROM Vendors WHERE Region = 'USA'", conn);
SAPAribaSourceDataAdapter da = new SAPAribaSourceDataAdapter(comm);
DataSet dataset = new DataSet();
da.Fill(dataset);
chart1.DataSource = dataset;
chart1.Series[0].XValueMember = "SMVendorID";
chart1.Series[0].YValueMembers = "Category";
// Insert code for additional chart formatting here.
chart1.DataBind();