Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →DataBind Zoom Data to the DevExpress Data Grid
Use the CData ADO.NET Provider for Zoom with the DevExpress Windows Forms and Web controls to provide Zoom data to a chart.
The ADO.NET Provider for Zoom by CData incorporates conventional ADO.NET data access components compatible with third-party controls. You can adhere to the standard ADO.NET data binding procedures to establish two-way access to real-time data through UI controls. This article will demonstrate the utilization of CData components for data binding with DevExpress UI Controls (Windows Forms and Web controls), specifically binding to a chart that visualizes live data.
Start by setting the Profile connection property to the location of the Zoom Profile on disk (e.g. C:\profiles\Zoom.apip). Next, set the ProfileSettings connection property to the connection string for Zoom (see below).
Zoom API Profile Settings
To authenticate to Zoom, you can use the OAuth standard to connect to your own data or to allow other users to connect to their data.
First you will need to create an OAuth app. To do so, navigate to https://marketplace.zoom.us/develop/create and click Create under the OAuth section. Select whether or not the app will be for individual users or for the entire account, and uncheck the box to publish the app. Give the app a name and click Create. You will then be given your Client Secret and Client ID
After setting the following connection properties, you are ready to connect:
- AuthScheme: Set this to OAuth.
- InitiateOAuth: Set this to GETANDREFRESH. You can use InitiateOAuth to manage the process to obtain the OAuthAccessToken.
- OAuthClientID: Set this to the OAuth Client ID that is specified in your app settings.
- OAuthClientSecret: Set this to the OAuth Client Secret that is specified in your app settings.
- CallbackURL: Set this to the Redirect URI you specified in your app settings.
Windows Forms Controls
The code below shows how to populate a DevExpress chart with Zoom data. The APIDataAdapter binds to the Series property of the chart control. The Diagram property of the control defines the x- and y-axes as the column names.
using (APIConnection connection = new APIConnection(
"Profile=C:\profiles\Zoom.apip;Authscheme=OAuth;OAuthClientId=your_client_id;OAuthClientSecret=your_client_secret;CallbackUrl=your_callback_url;InitiateOAuth=GETANDREFRESH")) {
APIDataAdapter dataAdapter = new APIDataAdapter(
"SELECT Id, JobTitle FROM MeetingRegistrants WHERE State = 'NC'", connection);
DataTable table = new DataTable();
dataAdapter.Fill(table);
DevExpress.XtraCharts.Series series = new DevExpress.XtraCharts.Series();
chartControl1.Series.Add(series);
series.DataSource = table;
series.ValueDataMembers.AddRange(new string[] { "JobTitle" });
series.ArgumentScaleType = DevExpress.XtraCharts.ScaleType.Qualitative;
series.ArgumentDataMember = "Id";
series.ValueScaleType = DevExpress.XtraCharts.ScaleType.Numerical;
chartControl1.Legend.Visibility = DevExpress.Utils.DefaultBoolean.False;
((DevExpress.XtraCharts.SideBySideBarSeriesView)series.View).ColorEach = true;
}
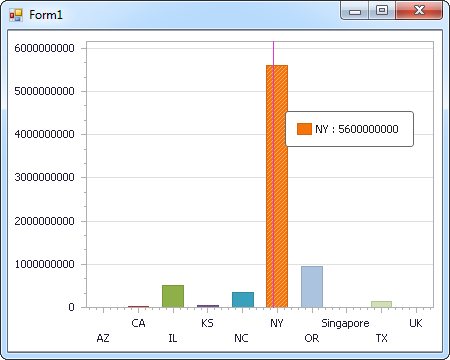
Web Controls
The code below shows how to populate a DevExpress Web control with Zoom data. The APIDataAdapter binds to the Series property of the chart; the Diagram property defines the x- and y-axes as the column names.
using DevExpress.XtraCharts;
using (APIConnection connection = new APIConnection(
"Profile=C:\profiles\Zoom.apip;Authscheme=OAuth;OAuthClientId=your_client_id;OAuthClientSecret=your_client_secret;CallbackUrl=your_callback_url;InitiateOAuth=GETANDREFRESH"))
{
APIDataAdapter APIDataAdapter1 = new APIDataAdapter("SELECT Id, JobTitle FROM MeetingRegistrants WHERE State = 'NC'", connection);
DataTable table = new DataTable();
APIDataAdapter1.Fill(table);
DevExpress.XtraCharts.Series series = new Series("Series1", ViewType.Bar);
WebChartControl1.Series.Add(series);
series.DataSource = table;
series.ValueDataMembers.AddRange(new string[] { "JobTitle" });
series.ArgumentScaleType = ScaleType.Qualitative;
series.ArgumentDataMember = "Id";
series.ValueScaleType = ScaleType.Numerical;
((DevExpress.XtraCharts.SideBySideBarSeriesView)series.View).ColorEach = true;
}
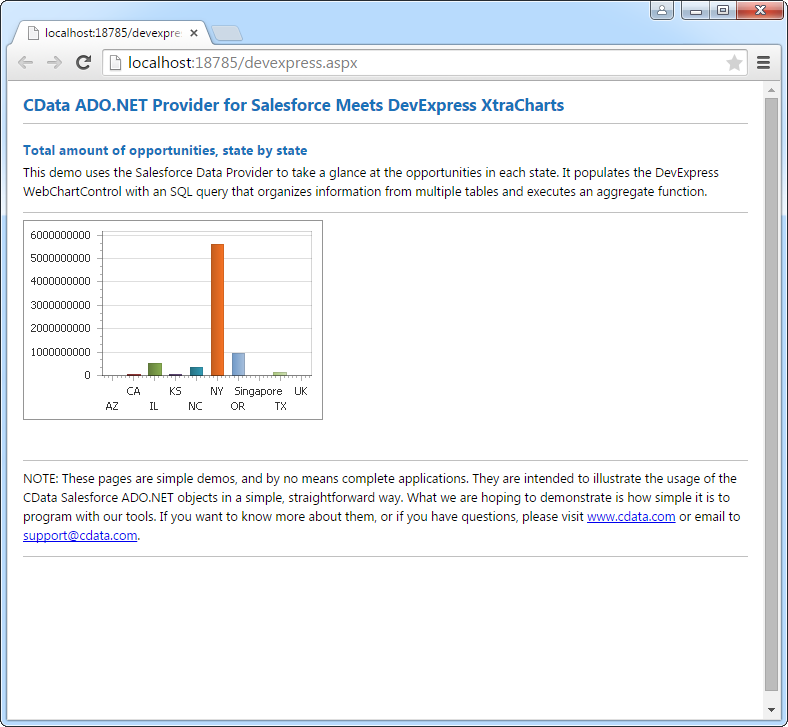