Discover how a bimodal integration strategy can address the major data management challenges facing your organization today.
Get the Report →Create Dynamic Zuora Grids Using the Infragistics XamDataGrid
Learn how you can connect Zuora to Infragistics XamDataGrid to build dynamic grids.
Using Infragistics WPF UI controls, you can build contemporary applications reminiscent of Microsoft Office for both desktop and touch-based devices. When coupled with the CData ADO.NET Provider for Zuora, you gain the capability to construct interactive grids, charts, and various other visual elements while directly accessing real-time data from Zuora data. This article will guide you through the process of creating a dynamic grid within Visual Studio using the Infragistics XamDataGrid control.
You will need to install the Infragistics WPF UI components to continue. Download a free trial here: https://www.infragistics.com/products/wpf.
Create a WPF Project
Open VisualStudio and create a new WPF project.
Add a TextBox for passing a SQL query to the CData ADO.NET Provider and a Button for executing the query.
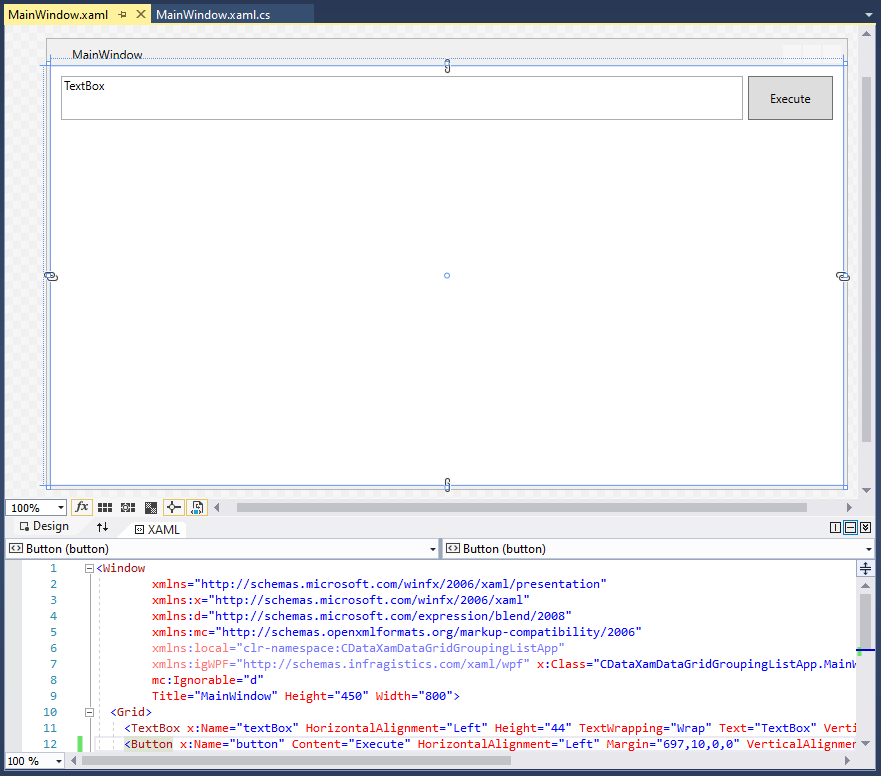
The XAML at this stage is as follows:
< Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:CDataXamDataGridGroupingListApp" xmlns:igWPF="http://schemas.infragistics.com/xaml/wpf" x:Class="CDataXamDataGridGroupingListApp.MainWindow" mc:Ignorable="d" Title="MainWindow" Height="450" Width="800"> < Grid> < TextBox x:Name="textBox" HorizontalAlignment="Left" Height="44" TextWrapping="Wrap" Text="TextBox" VerticalAlignment="Top" Width="682" Margin="10,10,0,0"/> < Button x:Name="button" Content="Execute" HorizontalAlignment="Left" Margin="697,10,0,0" VerticalAlignment="Top" Width="85" Height="44"/> < /Grid> < /Window>
Add and Configure a XamDataGrid
After adding the initial controls, add a XamDataGrid to the App. The component will appear in the Visual Studio toolbox.
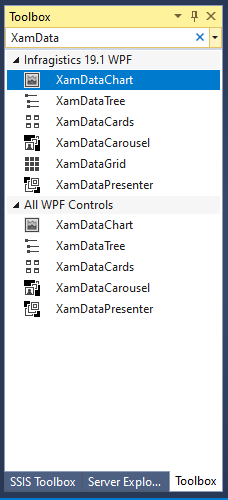
Arrange the component on the designer so that it is below the TextBox & Button and linked to the boundaries of the app.
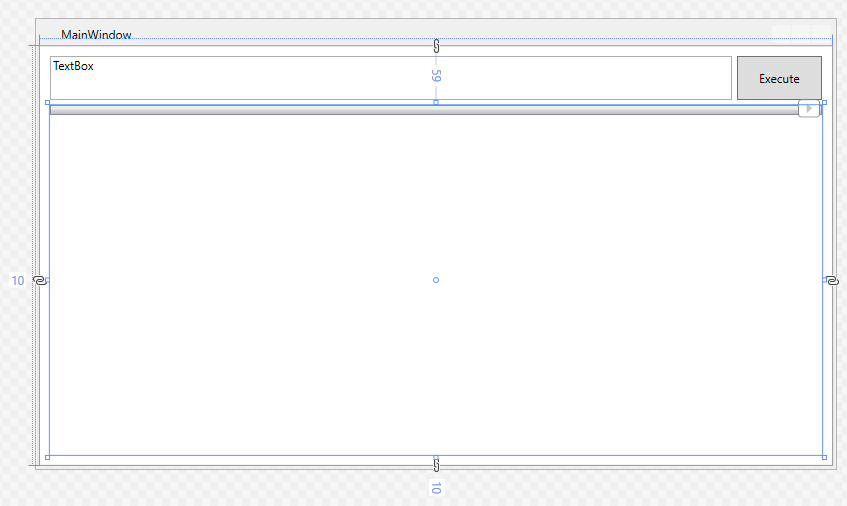
Once the XamDataGrid is placed, edit the XAML to set the XamDataGrid DataSource attribute to "{Binding}" and set the FieldSettings AllowRecordFiltering and AllowSummaries attributes to "true." Next, add an empty method as the Click event handler for the Button component. The XAML at this stage is as follows:
< Window xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:CDataXamDataGridGroupingListApp" xmlns:igWPF="http://schemas.infragistics.com/xaml/wpf" x:Class="CDataXamDataGridGroupingListApp.MainWindow" mc:Ignorable="d" Title="MainWindow" Height="450" Width="800"> < Grid> < TextBox x:Name="textBox" HorizontalAlignment="Left" Height="44" TextWrapping="Wrap" Text="TextBox" VerticalAlignment="Top" Width="682" Margin="10,10,0,0"/> < Button x:Name="button" Content="Execute" HorizontalAlignment="Left" Margin="697,10,0,0" VerticalAlignment="Top" Width="85" Click="Button_Click" Height="44"/> < igWPF:XamDataGrid Margin="10,59,10,10" DataSource="{Binding}"> < igWPF:XamDataGrid.FieldSettings> < igWPF:FieldSettings AllowSummaries="True" AllowRecordFiltering="True"/> < /igWPF:XamDataGrid.FieldSettings> < /igWPF:XamDataGrid> < /Grid> < /Window>
Connect to and Query Zuora
The last step in building our WPG App with a dynamic DataGrid is connecting to and querying live Zuora data. First add a reference to the CData ADO.NET Provider to the project (typically found in C:\Program Files\CData[product_name]\lib).
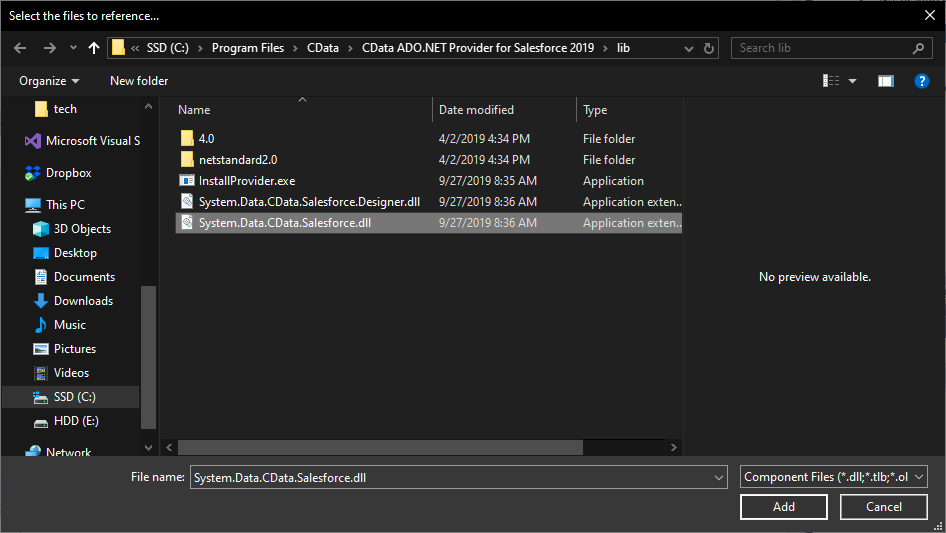
Next, add the Provider to the namespace, along with the standard Data library:
using System.Data.CData.Zuora; using System.Data;
Finally, add the code to connect to Zuora and query using the text from the TextBox to the Click event handler.
Zuora uses the OAuth standard to authenticate users. See the online Help documentation for a full OAuth authentication guide.
Configuring Tenant property
In order to create a valid connection with the provider you need to choose one of the Tenant values (USProduction by default) which matches your account configuration. The following is a list with the available options:
- USProduction: Requests sent to https://rest.zuora.com.
- USAPISandbox: Requests sent to https://rest.apisandbox.zuora.com"
- USPerformanceTest: Requests sent to https://rest.pt1.zuora.com"
- EUProduction: Requests sent to https://rest.eu.zuora.com"
- EUSandbox: Requests sent to https://rest.sandbox.eu.zuora.com"
Selecting a Zuora Service
Two Zuora services are available: Data Query and AQuA API. By default ZuoraService is set to AQuADataExport.
DataQuery
The Data Query feature enables you to export data from your Zuora tenant by performing asynchronous, read-only SQL queries. We recommend to use this service for quick lightweight SQL queries.
Limitations- The maximum number of input records per table after filters have been applied: 1,000,000
- The maximum number of output records: 100,000
- The maximum number of simultaneous queries submitted for execution per tenant: 5
- The maximum number of queued queries submitted for execution after reaching the limitation of simultaneous queries per tenant: 10
- The maximum processing time for each query in hours: 1
- The maximum size of memory allocated to each query in GB: 2
- The maximum number of indices when using Index Join, in other words, the maximum number of records being returned by the left table based on the unique value used in the WHERE clause when using Index Join: 20,000
AQuADataExport
AQuA API export is designed to export all the records for all the objects ( tables ). AQuA query jobs have the following limitations:
Limitations- If a query in an AQuA job is executed longer than 8 hours, this job will be killed automatically.
- The killed AQuA job can be retried three times before returned as failed.
private void Button_Click(object sender, RoutedEventArgs e) { //connecting to Zuora string connString = "OAuthClientID=MyOAuthClientId;OAuthClientSecret=MyOAuthClientSecret;Tenant=USProduction;ZuoraService=DataQuery;"; using (var conn = new ZuoraConnection(connString)) { //using the query from the TextBox var dataAdapter = new ZuoraDataAdapter(textBox.Text, conn); var table = new DataTable(); dataAdapter.Fill(table); //passing the DataRowCollection to the DataContext // for use in the XamDataGrid this.DataContext = table.Rows; } }
Run the Application
With the app fully configured, we are ready to display Zuora data in our XamDataGrid. When you click "Execute," the app connects to Zuora and submits the SQL query through the CData ADO.NET Provider.
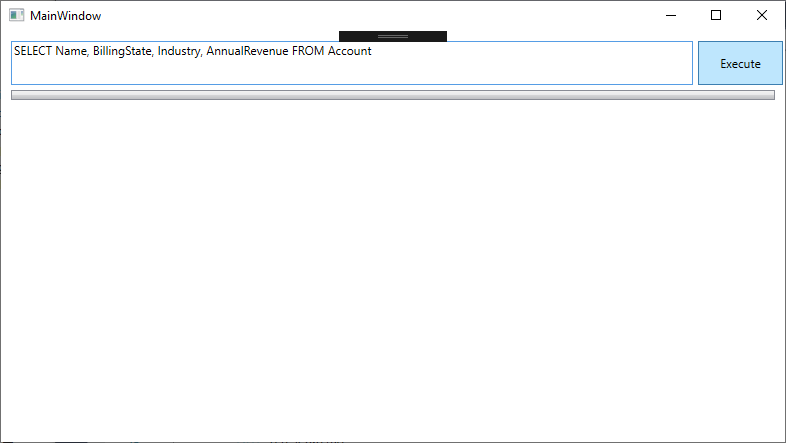
Live Zuora data is displayed in the grid.
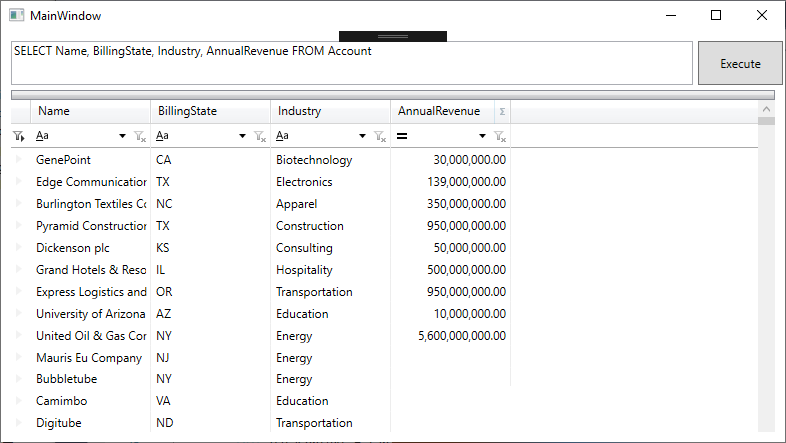
Group the data by dragging and dropping a column name into the header.
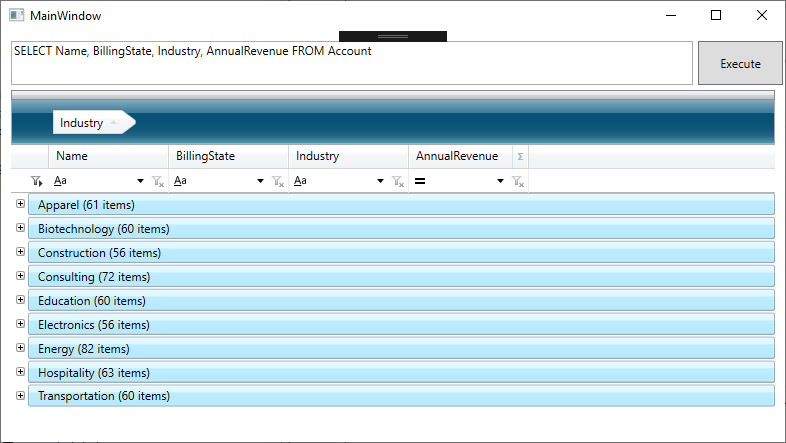
As you add groupings and filters, the underlying SQL query is submitted directly to Zuora, making it possible to drill down into live Zuora data to find only the specific information you need.
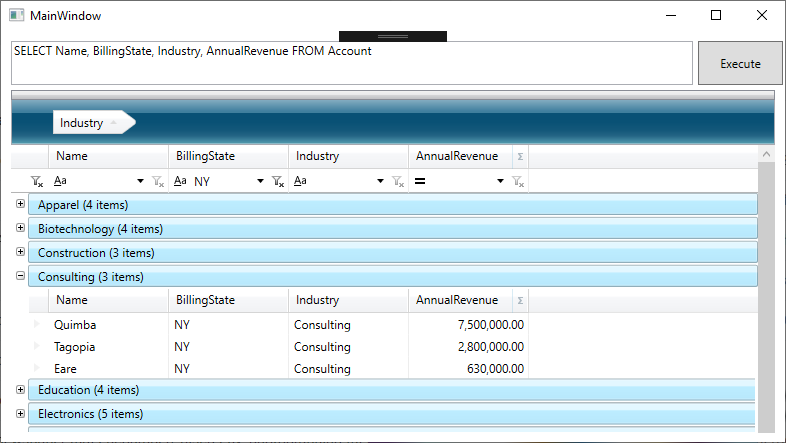
Free Trial & More Information
At this point, you have created a dynamic WPF App with access to live Zuora data. For more information, visit the CData ADO.NET Provider page. Download a free, 30-day trial and start working live Zuora data in apps built using the Infragistics UI controls today.